Week-11 Program-01
Due on 2024-04-11, 23:59 IST
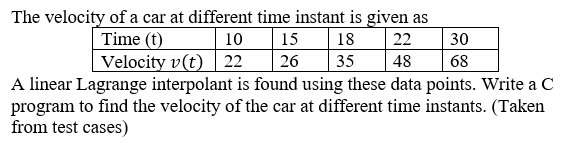
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 25 | The respective value of the variable v is: 56.42 | The respective value of the variable v is: 56.42 | Passed |
Test Case 2 | 16 | The respective value of the variable v is: 28.74 | The respective value of the variable v is: 28.74 | Passed |
Week-11 Program-02
Due on 2024-04-11, 23:59 IST

Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 0
1 | The integral is: 0.335000 | The integral is: 0.335000 | Passed |
Test Case 2 | 1
3 | The integral is: 8.680000 | The integral is: 8.680000 | Passed |
Week-11 Program-03
Due on 2024-04-11, 23:59 IST
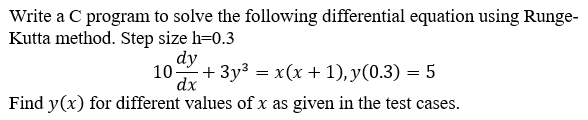
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 0.9 | y=1.777165 | y=1.777165 | Passed |
Test Case 2 | 1.2 | y=1.468128 | y=1.468128 | Passed |
Week-11 Program-04
Due on 2024-04-11, 23:59 IST
Write a C program to check whether the given input number is Prime number or not using recursion. So, the input is an integer and output should print whether the integer is prime or not. Note that you have to use recursion.
Public Test Cases | Input | Expected Output | Actual Output | Status |
---|---|---|---|---|
Test Case 1 | 13 | 13 is a prime number | 13 is a prime number\n
| Passed after ignoring Presentation Error |
Test Case 2 | 40 | 40 is not a prime number | 40 is not a prime number\n
| Passed after ignoring Presentation Error |
No comments